The Art of Debugging: Full Stack Java Developer's Toolkit
The Art of Debugging A comprehensive toolkit for Full Stack Java Developers. Learn essential debugging techniques and tools to streamline your Java development.
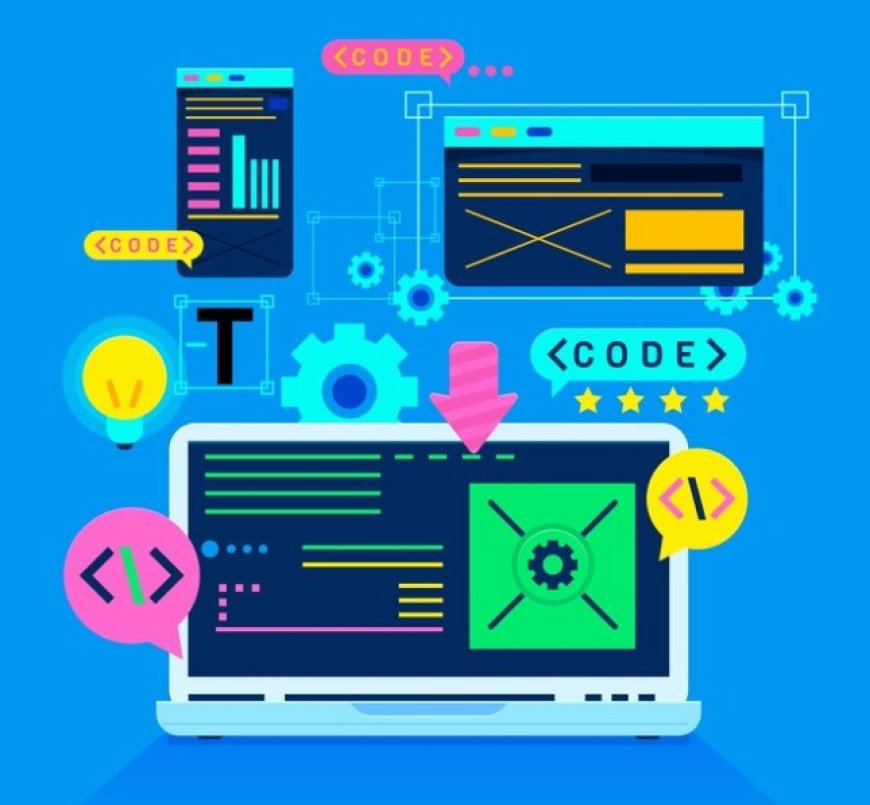
The introduction sets the stage by emphasizing the pivotal role of debugging in software development. It highlights how effective debugging ensures the integrity and functionality of a program. The section also provides a brief overview of the debugging toolkit, giving readers a glimpse into the essential tools and techniques they will delve into, equipping them with the skills to identify and rectify errors efficiently. This foundational knowledge sets the tone for the subsequent chapters, establishing the significance of adept debugging practices in the realm of software development.
Understanding the Java Debugger
Setting up a robust debugging environment is crucial for efficient troubleshooting. In this section, we'll explore how to configure Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse for Java debugging. We'll cover setting breakpoints, configuring debugging options, and connecting to your Java application.
To become proficient in debugging, you need to master fundamental commands and techniques. We'll delve into the basics of using the Java debugger, such as stepping through code, inspecting variables, and evaluating expressions. These skills are essential for pinpointing and resolving issues in your Java applications.
Debugging often involves scrutinizing data structures and variables. In this part, we'll learn how to effectively examine and manipulate data during debugging sessions. We'll explore techniques for inspecting complex data structures, arrays, and objects, helping you gain insights into how your Java program processes information and where potential problems may arise.
Debugging Front-End Java
Debugging Java applets and JavaFX applications:
Debugging front-end Java applications like applets and JavaFX requires specialized techniques. Developers will learn how to set up debugging environments for these app types within their integrated development environments (IDEs). They'll explore techniques for stepping through code, inspecting variables, and identifying issues unique to graphical user interfaces.
Handling client-side issues with browser developer tools:
In the world of web development, client-side debugging is crucial. This section delves into how Java developers can leverage browser developer tools like those in Chrome or Firefox to diagnose and resolve front-end issues. Topics include inspecting HTML, CSS, and JavaScript, as well as leveraging browser consoles for error tracking.
Cross-browser compatibility debugging:
Cross-browser compatibility is a common challenge in web development. Here, developers will discover strategies and tools to identify and address issues that arise when their Java-based web applications run on different browsers. Techniques for testing, debugging, and ensuring consistent functionality across various browsers will be explored in detail.
Debugging Back-End Java
Debugging the back-end of Java applications is crucial for ensuring their reliability and performance. This section will delve into the intricacies of identifying and resolving issues in server-side code, especially when dealing with technologies like Servlets, JavaServer Pages (JSP), and database interactions using Java Database Connectivity (JDBC).
You'll learn how to effectively trace the flow of server-side requests, set breakpoints, and inspect variables. Handling database-related bugs and optimizing SQL queries for better performance will also be explored. Additionally, debugging RESTful APIs and microservices will be covered, providing you with the tools to ensure your back-end Java components function seamlessly. Debugging in this context is essential for maintaining a robust and efficient server-side infrastructure.
Debugging Multi-Tier Applications
Debugging multi-tier applications involves addressing complexities that arise from the interaction between various layers of the application stack. It requires a holistic approach to identify and resolve issues that may span from the front-end user interface to the back-end server and database. This section will delve into strategies for debugging communication between different tiers, including techniques for diagnosing and rectifying issues that can impact the seamless flow of data and functionality between these layers. Additionally, we'll explore methods for detecting and mitigating performance bottlenecks that may arise in multi-tier architectures, ensuring that the application runs efficiently and smoothly across all its components.
Debugging Java in Production
Debugging in a live environment presents unique challenges and risks. Developers need strategies to diagnose issues without disrupting service. This section explores techniques like A/B testing, canary releases, and feature flags to safely test changes. It also covers strategies for error recovery and minimizing downtime.
Monitoring and logging are vital for debugging in production. We delve into setting up comprehensive monitoring tools and implementing effective logging practices. Techniques like log aggregation, real-time monitoring, and alerting systems are discussed to identify and react to issues proactively.
Security and scalability concerns are paramount in a production environment. We address techniques for debugging security vulnerabilities, such as penetration testing and threat modeling. Additionally, we explore strategies for scaling applications, including load testing, horizontal scaling, and capacity planning, while keeping an eye on potential bottlenecks and performance optimizations.
Advanced Debugging Techniques
-
Remote Debugging and Distributed Systems: This section delves into the complexities of debugging in distributed environments. You'll learn how to set up and utilize remote debugging tools to trace issues across multiple servers or microservices. Understanding how to diagnose problems in distributed systems is crucial for modern full-stack Java developers.
-
Profiling and Performance Tuning: Profiling tools are essential for optimizing code performance. Here, you'll explore various profiling techniques and tools to identify bottlenecks, memory leaks, and resource-intensive operations. This knowledge empowers you to fine-tune your Java applications for optimal efficiency.
-
Debugging Tools and Third-Party Libraries: Discover a range of debugging tools and third-party libraries that can streamline your debugging process. We'll explore how to leverage these tools effectively to troubleshoot complex issues and make your debugging workflow more efficient. Additionally, we'll discuss best practices for integrating third-party libraries without introducing new bugs.
These advanced debugging techniques will equip you with the skills needed to tackle complex Java debugging scenarios, ensuring the robustness and performance of your full-stack Java applications.
Debugging Best Practices
Documentation and Code Organization for Easier Debugging
Effective documentation and well-organized code are foundational for streamlined debugging. Developers should maintain clear and up-to-date comments, function descriptions, and variable naming conventions. Code that follows a consistent structure and adheres to coding standards is easier to read and debug. Comprehensive documentation helps both individual developers and team members understand the code's purpose and logic, making it simpler to pinpoint and resolve issues efficiently.
Collaborative Debugging in a Team
Debugging often involves collaboration within development teams. Developers should establish clear communication channels to share insights, findings, and potential solutions. Collaborative tools and practices, such as version control systems and code reviews, facilitate efficient debugging in a team environment. Effective teamwork can lead to faster issue resolution and improved code quality.
Continuous Improvement and Post-Mortem Analysis
Debugging is an ongoing learning process. Teams should regularly assess their debugging practices and seek opportunities for improvement. Conducting post-mortem analyses after resolving critical issues can reveal valuable insights into the root causes and ways to prevent similar problems in the future. Embracing a culture of continuous improvement ensures that debugging skills evolve alongside software development practices, enhancing overall software quality and reliability.
Troubleshooting Common Java Issues
Java developers frequently encounter common errors such as NullPointerExceptions, ArrayIndexOutOfBoundsExceptions, and ClassCastException. This section will provide insights into identifying the root causes of these issues and offering practical solutions. It will also address common runtime exceptions and how to handle them gracefully. Additionally, it will cover common compile-time errors and debugging techniques to resolve them effectively.
Effective debugging goes beyond knowing error messages and stack traces. This part will provide valuable tips for efficient issue resolution. It will emphasize the importance of systematic debugging, including step-by-step code inspection, using breakpoints judiciously, and utilizing logging effectively. Moreover, it will stress the significance of isolating and reproducing issues, leveraging version control for tracking changes, and seeking help from the developer community when needed.
The Art of Debugging: Full Stack Java Developer's Toolkit equips developers with the essential skills and tools for effective debugging in Java-based applications. Throughout the book, we have explored fundamental debugging concepts, from setting up environments to handling complex issues in both front-end and back-end Java development. It is crucial to remember that debugging is an ongoing journey. Continuous learning and improvement are encouraged to stay at the forefront of this critical aspect of software development. Mastery of debugging is key to building robust and reliable Java applications.