Python - An Introduction to Programming and Beyond
Explore the world of Python programming in this comprehensive guide. Learn Python basics and advance into the realm of coding and beyond.
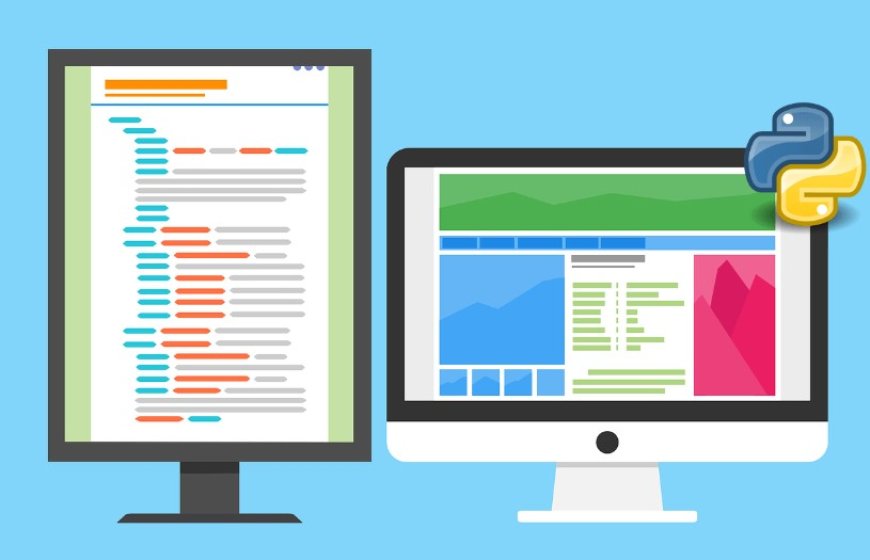
Python is a versatile and beginner-friendly programming language, widely used for various applications, from web development to data analysis and artificial intelligence. Its clear and concise syntax makes it easy to read and write code, making it an ideal choice for both novices and experienced developers. With an extensive standard library and a vast ecosystem of third-party packages, Python empowers developers to build efficient and powerful solutions quickly. Whether you're a programmer starting your journey or an experienced developer exploring new possibilities, Python offers an excellent foundation for your coding endeavors.
What is Python?
Python is a high-level, interpreted, and general-purpose programming language known for its simplicity and readability. It was created by Guido van Rossum and first released in 1991. Python's design philosophy emphasizes code readability, with a clear and expressive syntax that allows developers to write programs with fewer lines of code compared to other languages. It is widely used in web development, data science, automation, scripting, and more, making it a popular choice for beginners and experienced programmers alike.
Python Basics
Python Basics covers fundamental concepts that serve as building blocks for writing Python code.
Python syntax and structure: Python's syntax is clear and straightforward, emphasizing indentation to define blocks of code. It uses colons and whitespace to create structured code blocks, making it easy to read and understand.
Variables and data types: In Python, variables store data of different types, such as integers, floating-point numbers, strings, lists, tuples, and dictionaries. It is a dynamically typed language, allowing variables to change data types during runtime.
Operators and expressions: Python supports a variety of operators for arithmetic, comparison, logical operations, and more. Expressions are combinations of variables, values, and operators, evaluated to produce results.
Control flow statements (if, else, loops): Control flow statements allow programmers to control the order of execution in a program. "if" and "else" statements enable conditional execution, while loops, like "for" and "while," help execute code repetitively based on specific conditions.
Mastering these Python Basics provides a solid foundation for understanding and writing more complex programs in Python.
Data Structures in Python
Data Structures in Python are essential for organizing and storing data efficiently. Python offers several built-in data structures, including lists, tuples, sets, and dictionaries. Lists are versatile and mutable, allowing ordered collections of elements. Tuples are similar to lists but immutable, meaning their elements cannot be changed after creation. Sets are unordered collections of unique elements, useful for mathematical operations like unions and intersections. Dictionaries store data in key-value pairs, enabling fast retrieval and modification of data based on keys. Understanding and utilizing these data structures efficiently enhances the power and flexibility of Python programming.
Functions and Modules
Functions in Python:
-
Functions are blocks of organized, reusable code.
-
They are defined using the "def" keyword followed by a function name and parameters.
-
Functions can return values using the "return" statement.
-
Parameters can have default values, making them optional when calling the function.
-
"docstrings" can be added to provide documentation for the function's purpose.
Modules in Python:
-
Modules are files containing Python code and functions that can be imported into other programs.
-
They help organize code into separate files for better maintainability.
-
Importing modules is done using the "import" statement.
-
Python's standard library provides a wide range of built-in modules for various functionalities.
-
Custom modules can be created to group related functions and variables.
Object-Oriented Programming (OOP) in Python
Object-Oriented Programming (OOP) in Python is a programming paradigm that focuses on creating objects that encapsulate data and behavior. Python supports OOP principles, including classes and objects. Classes act as blueprints for creating objects, defining their attributes (data) and methods (functions). Objects are instances of classes, allowing multiple copies of the same structure with distinct data. OOP promotes code reusability, modularity, and a clear separation of concerns, making Python a versatile language for building complex and scalable applications.
File Handling and Input/Output
File Handling and Input/Output are essential aspects of Python programming for interacting with external data and user input.
-
Reading and writing to files: Python provides built-in functions to read and write data from/to files. The "open()" function is used to open files, and "read()" and "write()" methods enable reading and writing data, respectively.
-
Working with CSV and JSON files: Python's "csv" module facilitates working with comma-separated values (CSV) files, common for data storage. The "json" module enables reading and writing JSON (JavaScript Object Notation) data, a popular format for data interchange.
-
Command-line arguments and user input: Python can accept command-line arguments when executing a script. The "sys" module allows accessing command-line arguments. Additionally, Python's "input()" function allows developers to interact with users, prompting them for input during program execution.
Understanding file handling and input/output in Python empowers developers to handle external data efficiently and create interactive applications with user input capabilities.
Exception Handling
Exception Handling is a critical aspect of Python programming to manage errors and ensure robust code execution.
Exceptions are unexpected events that occur during program execution, disrupting the normal flow. Common errors include "ZeroDivisionError," "TypeError," and "FileNotFoundError." Understanding exceptions helps developers identify potential issues and handle them gracefully.
Python's "try-except" mechanism allows developers to catch and handle exceptions gracefully. Code that might raise exceptions is placed inside a "try" block, and potential exception handlers are defined in "except" blocks.
Python allows the creation of custom exception classes to represent specific error scenarios in applications. By defining custom exception classes, developers can provide meaningful error messages and more precise handling of exceptional situations.
Effectively implementing exception handling ensures that Python programs can gracefully recover from errors, provide helpful feedback to users, and maintain stability during execution.
Working with Libraries and Packages
Working with Libraries and Packages in Python is a fundamental aspect of leveraging the language's vast ecosystem and enhancing development productivity.
Python's standard library already contains a wide range of modules and packages that provide various functionalities for tasks such as file handling, networking, and data manipulation. However, the true power lies in the extensive collection of third-party libraries and packages created by the Python community. These external libraries address specific needs, including data science, web development, machine learning, and more.
To use external libraries, developers can use Python's package manager, "pip," to install them easily. Once installed, importing the libraries into the code allows access to their functions and tools, significantly accelerating the development process and extending Python's capabilities beyond its core features.
Introduction to Web Development with Python
Introduction to Web Development with Python involves using web frameworks and building web applications and APIs.
-
Web frameworks (e.g., Django, Flask): Python offers powerful web frameworks like Django and Flask, which streamline web development by providing essential tools and structures. Django is a full-featured framework suitable for complex applications, while Flask is a micro-framework designed for simplicity and flexibility. These frameworks handle routing, database interactions, and template rendering, allowing developers to focus on application logic.
-
Creating web applications and APIs: With Python web frameworks, developers can create dynamic web applications and APIs efficiently. Web applications provide interactive user interfaces, while APIs enable data exchange between different systems. By combining Python's versatility and the capabilities of web frameworks, developers can deliver robust and scalable web solutions.
Introduction to Data Science and Machine Learning with Python
Data Science in Python involves using libraries like NumPy and Pandas for data manipulation and exploration, Matplotlib and Seaborn for data visualization, and Scikit-learn for machine learning tasks. Python's data science ecosystem provides a seamless workflow for extracting insights and making data-driven decisions.
Machine Learning with Python enables the development of intelligent systems capable of learning from data. Scikit-learn offers a wide range of algorithms for classification, regression, clustering, and more. By leveraging Python's simplicity and the extensive machine learning libraries, developers can create models that solve complex real-world problems.
Best Practices and Tips
-
Use meaningful variable names and follow PEP 8 style guidelines for code readability.
-
Comment your code to explain its purpose and logic clearly.
-
Avoid using global variables; prefer passing variables as function arguments.
-
Write modular code with functions to improve code reusability.
-
Handle exceptions gracefully with try-except blocks.
-
Use list comprehensions and generator expressions for efficient data processing.
-
Leverage Python's built-in data structures (lists, dictionaries, sets) for convenient data handling.
-
Make use of virtual environments to manage project dependencies and avoid conflicts.
-
Optimize performance with appropriate data structures and algorithm choices.
-
Take advantage of Python's standard library and third-party packages to avoid reinventing the wheel.
Python is a powerful and versatile programming language that offers simplicity, readability, and a vast ecosystem of libraries and frameworks. From its user-friendly syntax to its applications in web development, data science, and machine learning, Python continues to be a popular choice for developers worldwide. Embracing Python's best practices and continuous learning from the thriving community ensure a successful and enjoyable coding experience, making Python a go-to language for a wide range of projects and industries.